min()
is a built-in function in Python 3. It returns the smallest item in an iterable, or the smallest of two or more arguments.
Arguments
Number-theoretic and representation functions¶ math.ceil(x)¶ Return the ceiling of x, the smallest. Python program that uses max, min on string list values = 'cat', 'bird', 'apple' # Use max on the list of strings. Result = max (values) print ('MAX', values, result) # Use min. Result = min (values) print ('MIN', values, result) MAX 'cat', 'bird', 'apple' cat MIN 'cat', 'bird', 'apple' apple.
Python Math Minutes
This function takes two or more numbers or any kind of iterable as an argument. While giving an iterable as an argument we must make sure that all the elements in the iterable are of the same type. This means that we cannot pass a list which has both string and integer values stored in it.
Valid Arguments:
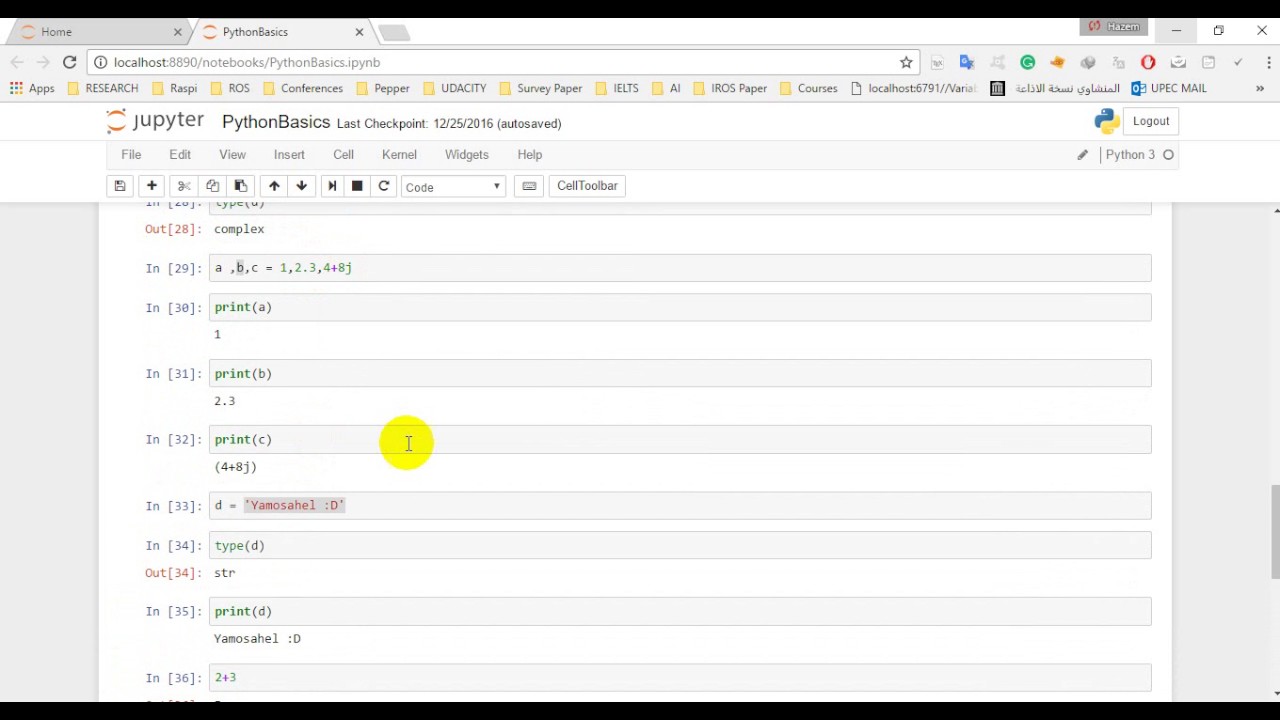
Invalid Arguments:
Return Value
The smallest item in the iterable is returned. If two or more positional arguments are provided, the smallest of the positional arguments is returned. If the iterable is empty and default is not provided, a ValueError is raised.
Code Sample
Get the minimum value of column in python pandas : In this tutorial we will learn How to get the minimum value of all the columns in dataframe of python pandas. How to get the minimum value of a specific column or a series using min() function.
Syntax of Pandas Min() Function:
axis | 0 – Rows wise operation |
1- Columns wise operation | |
skipna | Exclude NA/null values when computing the result |
level | If the axis is a Multi index (hierarchical), count along a particular level, collapsing into a Series |
numeric_only | Include only float, int, boolean columns. If None, will attempt to use everything |
We will looking at an example on
- How to get Column wise minimum value of all the column.
- Get minimum value of a specific column by name
- Get minimum value of series in pandas python
- Get minimum value of a specific column by index
Create Dataframe:
So the resultant dataframe will be
Get the minimum value of all the column in python pandas:
This gives the list of all the column names and its minimum value, so the output will be
Get the minimum value of a specific column in python pandas:
Example 1:
This gives the minimum value of column Age so the output will be
Example 2:
This gives the minimum value of column Name so the output will be
Get the minimum value of a specific column in pandas by column index:
df.iloc[] gets the column index as input here column index 1 is passed which is 2nd column (“Age” column) , minimum value of the 2nd column is calculated using min() function as shown.
Get Minimum value of the series in pandas :
Lastly we would see how to calculate the minimum value of a series in pandas by using min() function . First lets create a series of alphabets as shown below
created series is
Python Math Operators
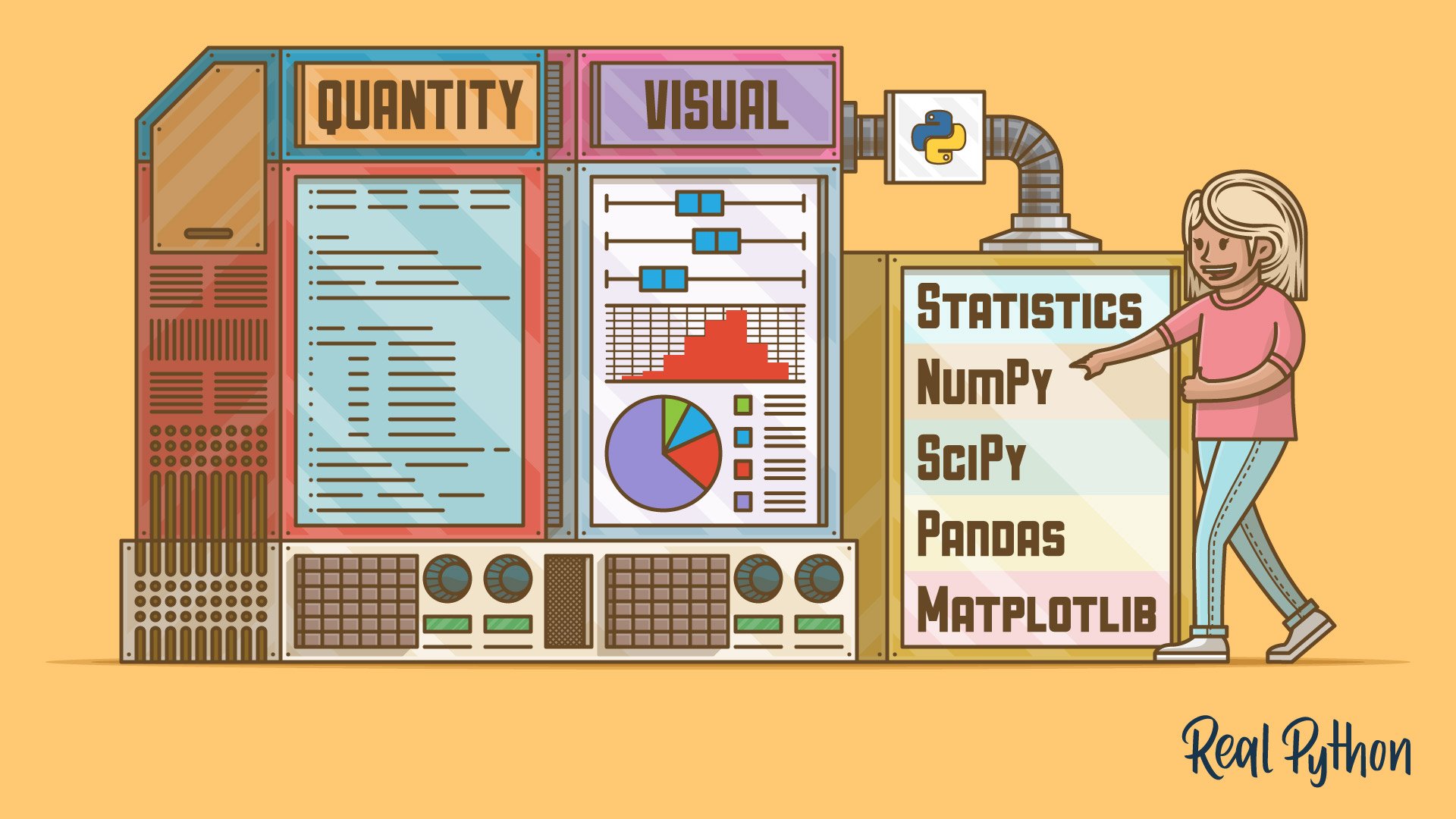
Python Math Plus Minus
Minimum value of a series is calculated using series.min() function as shown below
Python Math Min
so the resultant min value of the series is
